Best practices for using Playwright Fixtures in end-to-end testing
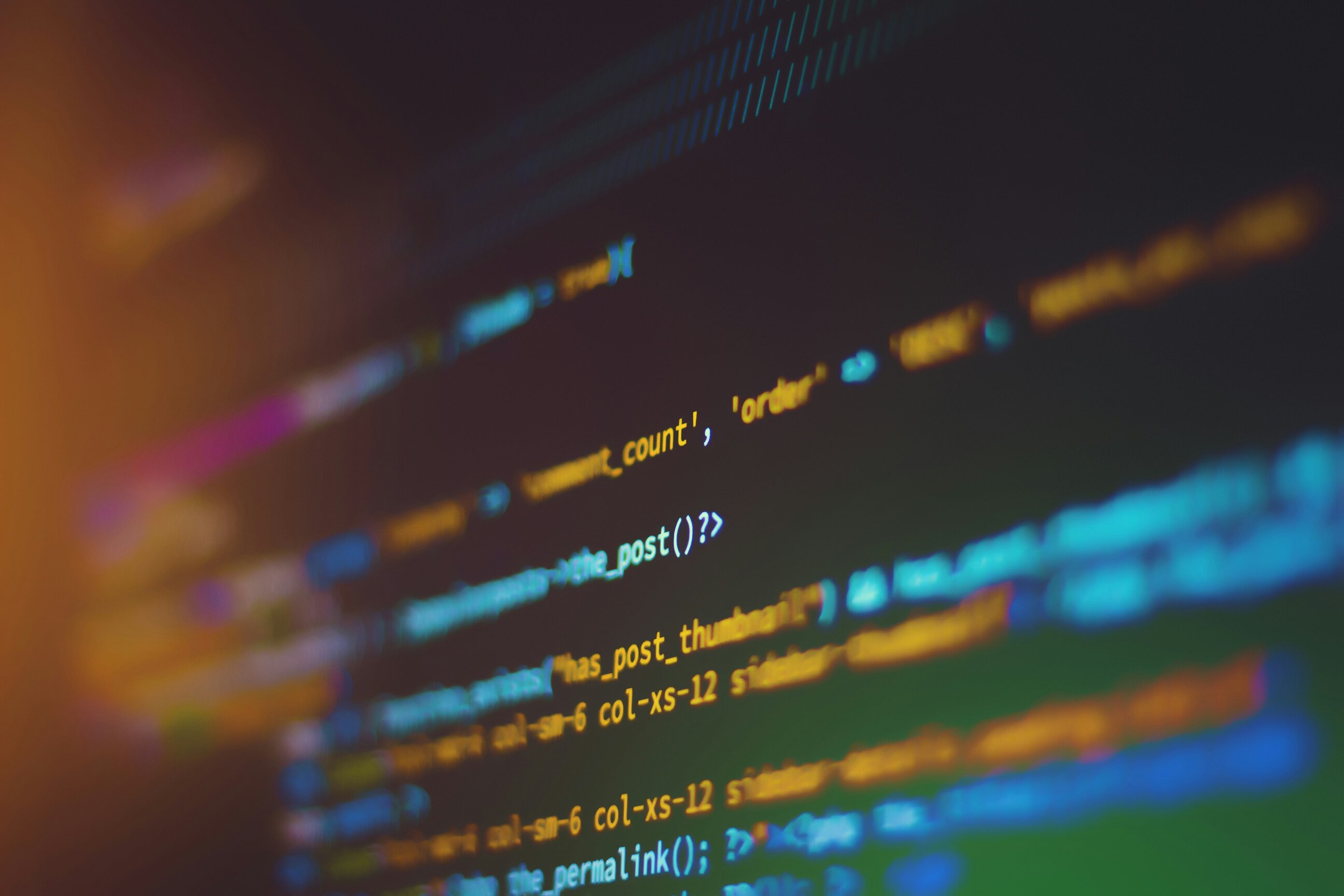
End-to-end (E2E) testing is vital for ensuring that applications deliver the intended functionality to users. Playwright, a robust framework for automating browsers, offers tools to create reliable and maintainable tests. Among its most valuable features are fixtures, which streamline test setups and enhance reusability. This article explores essential practices for writing effective Playwright tests, focusing on user interactions, locator strategies, test isolation, naming conventions, and CI/CD integration.
1. Execute user interactions with an application
Testing real-world user interactions ensures your application works as intended. Focus on covering the most important workflows and securing the application against potential vulnerabilities.
Test for happy paths
Happy paths are the primary workflows users follow, such as signing up, logging in, or completing a purchase. These tests confirm that the most common scenarios function seamlessly.
Example:
Test that a user can successfully log in and navigate to their dashboard.
Test for regression and vulnerabilities
- Regression testing: ensure that new changes don’t break existing functionality. For example, validate that the checkout process works after introducing new payment methods.
- Vulnerability testing: verify security measures for frontend functionality, such as access controls. Ensure users with specific privileges can access only the intended pages. Avoid testing API security, which is typically a backend responsibility.
Example:
Test that an admin can access the settings page, while a regular user cannot.
2. Using locators effectively
Locators are the backbone of Playwright tests, enabling interaction with elements on a page. Proper locator strategies make tests resilient and maintainable.
Use locators based on accessibility
Accessibility attributes, such as aria-label
and role
, create stable locators and align with web standards. They also contribute to building inclusive applications.
Example:
const searchBox = page.getByPlaceholder('Search');
await searchBox.fill('Playwright');
Leverage Playwright-provided locators
Playwright’s built-in locators, like getByRole
and getByText
, include features like auto-waiting and retry-ability, reducing flaky tests caused by dynamic content.
Example:
const submitButton = page.getByRole('button', { name: 'Submit' });
await submitButton.click();
Chain locators for clarity
Locator chaining helps target nested elements while keeping tests readable.
Example:
const menuOption = page.getByRole('menu').getByText('Settings');
await menuOption.click();
Extract locators to variables
Extracting frequently used locators into variables avoids repetition and improves readability.
Example:
const searchInput = page.getByPlaceholder('Search');
await searchInput.fill('Test Query');
3. Tests should be as isolated as possible
Test isolation is critical for ensuring that test results are reliable and not influenced by other tests. A common mistake is creating test data in one test and deleting it in another. This approach is fragile and prone to failure when tests run in parallel. If tests must depend on shared data, they should be configured to run sequentially in a single thread. Below is an example configuration for running tests in order:
Sequential test configuration:
{
"projects": [
{
"name": "sequential-tests",
"testMatch": "tests/sequential/*.spec.js",
"fullyParallel": false
}
]
}
Or for single file by using:
test.describe.configure({ mode: 'serial' });
Whenever possible, avoid such dependencies by ensuring each test manages its own setup and teardown.
Reusability for consistency
Repeatedly writing the same code for setup or interactions is normal during test development. However, this approach increases maintenance effort when changes occur. Extracting such logic into reusable functions ensures consistency and simplifies updates by centralizing changes in a single location.
Using hooks for reusability
For repetitive setups that should occur before or after every test, use beforeEach
and afterEach
hooks. This method ensures uniform execution but is limited to scenarios where all tests require the same setup.
Example:
test.beforeEach(async ({ page }) => {
await page.goto('/login');
await page.fill('#username', 'admin');
await page.fill('#password', 'password123');
await page.click('button[type="submit"]');
});
Page Object Model (POM) for flexibility
For scenarios requiring reusable logic in specific parts of a test or across different test files, adopting the Page Object Model (POM) pattern is highly effective. POM organizes your application interactions into dedicated classes, making tests cleaner and easier to maintain.
Example:
Create a LoginPage
object to handle login interactions:
class LoginPage {
constructor(page) {
this.page = page;
this.usernameInput = page.locator('#username');
this.passwordInput = page.locator('#password');
this.submitButton = page.locator('button[type="submit"]');
}
async login(username, password) {
await this.usernameInput.fill(username);
await this.passwordInput.fill(password);
await this.submitButton.click();
}
}
// Usage in tests
test('admin logs in', async ({ page }) => {
const loginPage = new LoginPage(page);
await loginPage.login('admin', 'password123');
});
This approach simplifies updates and ensures consistent behavior across tests.
Efficient handling of local storage for login
For login scenarios, a more efficient approach involves using fixtures to save the logged-in state in local storage. This eliminates the need to perform a login operation in every test.
Steps to implement:
- Run a login test that saves the local storage state after logging in.
- Save the state to a file using
storageState
. - Reuse this file across tests for users with the same privileges.
Example:
// test.setup.ts
test('authenticate as USER', async ({ page }) => {
// login flow
await page.context().storageState({ path: ‘<path/to/file.json>’})
})
playwright.config.ts
projects: [
{
name: 'setup',
testMatch: /.*\.setup\.ts/,
use: {
bypassCSP: true,
launchOptions: {
args: ['--disable-web-security'],
},
},
},
Or you can use it in tests:test('some test', async ({ browser }) => {
const authorizedContext = await browser.newContext({
storageState: ‘<path/to/file.json>’,
})
const authPage = await authorizedContext.newPage()
await authPage.goto('/')
…
For different user roles, create separate local storage files (admin.json
, editor.json
, etc.) and load them selectively.
Combining with POM:
You can integrate this approach with POM by initializing specific states in your page objects, ensuring both reusability and clean test design.
Summary
- Avoid test dependencies by ensuring each test manages its setup and teardown independently. If unavoidable, configure them to run sequentially.
- Use hooks (
beforeEach
,afterEach
) for uniform setups across tests. - Adopt the Page Object Model (POM) pattern to centralize and reuse logic for interactions.
- Save and reuse local storage states for login scenarios to reduce redundancy and improve efficiency. Combine this with POM for maximum flexibility.
By following these practices, you’ll create isolated, maintainable, and robust tests that are easier to adapt as your application evolves.
4. Test naming
A good naming convention is crucial for test maintainability and debugging.
Best practices for test naming
- Be descriptive: include the “path” and scenario in the name. Example:
@login @admin admin navigates to settings page
. - Be consistent: maintain uniform naming conventions throughout your test suite.
- Avoid overly short names: clarity is more important than brevity.
Use tags for test organization
Tags allow you to group and run specific tests. For example:
npx playwright test --grep @login --debug
Tags can also be combined to filter tests for complex scenarios.
5. Running tests in CI/CD pipelines
Integrating Playwright tests into CI/CD workflows ensures that changes are continuously validated.
Key practices:
- Run isolated and stable tests to prevent false negatives.
- Leverage Playwright’s debugging tools, such as traces and screenshots, to troubleshoot issues in CI environments.
- Use tags to run only relevant tests during specific pipeline stages.
Example:
Run tagged tests as part of a deployment process:
npx playwright test --grep @critical
Conclusion
By focusing on user interactions, adopting efficient locator strategies, isolating tests, and maintaining clear naming conventions, you can build a robust and maintainable Playwright test suite. Integrating these tests into CI/CD pipelines ensures continuous quality assurance. Begin implementing these practices today to streamline your testing workflows and enhance your application’s reliability.
More Articles
Our team of experts is ready to partner with you to drive innovation, accelerate business growth, and achieve tangible results.
If you’re wondering how to make IT work for your business
let us know to schedule a call with our sales representative.